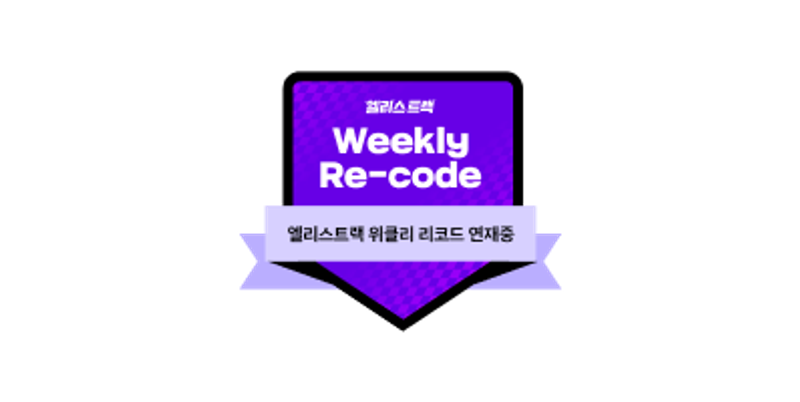
Mongodb 란?
- DataBase(하나 이상의 Collection을 가질 수 있는 저장소) →
Collection( 하나이상의 Document가 저장되는 공간) →
Document(저장되는 자료 SQL에서 row와 유사하지만 구조제약 없이 유연하게 저장가능) - Mongodb 는 비정형화된 데이터들을 저장하는 데이터베이스입니다.
- NoSQL 중에서 자주 사용하는 DB 이며 512MB 까지는 무료로 사용할 수 있습니다.
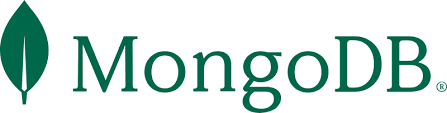
Mongoose 란?
- Mongoose 는 Mongodb 에서 schema를 보다 쉽게 관리할 수 있으며 Mongodb와의 연결 상태를 관리해주며 Populate를 사용하게 해주는 ODM ( Object Data Mapping) 입니다.
- Mongoose 를 사용하기 위해서는 패키지를 설치하여 사용합니다.
npm install mongoose
oryarn add mongoose
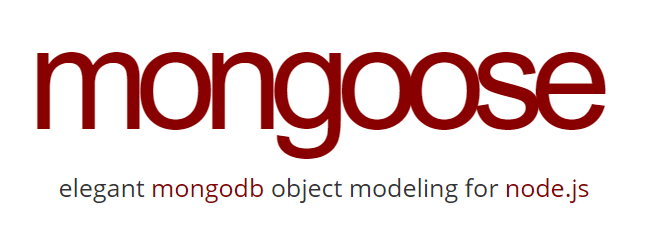
Mongoose 사용법
Mongoose 를 아래에 스키마와 모델을 생성하였다고 생각하고 CRUD 작업을 해보겠습니다.
const mongoose = require('mongoose');
const userSchema = mongoose.Schema({
name: String,
age: Number
})
const userModel = mongoose.model('users',userSchema);
Create (생성)
// 객체 생성을 통한 document 생성
const users = new userModel({ name:'tony', age: 23 })
await users.save();
// create 메서드 사용하여 document 생성
userModel.create({name: 'tony', age: 23})
Read (조회)
const users = await userModel.find({}); // 해당 collection 모두 조회
const user = await userModel.findOne({name: 'tony'}) // 해당 collection 에서 name 이 tony를 조회
const user = await userModel.findById(id) // _id를 기준으로 하나의 document를 반환
Update (변경)
const user = await userModel.update({name: 'tony'}, {name: 'william'}) // name이 tony 인 것을 wiliiam으로 변경!!
const user = await userModel.findByIdUpdate(id, {name: 'tony'}) // id에 해당하는 document를 찾아 name을 tony로 변경
const user = await userModel.findOneAndUpdate({ name: 'tony' }, // 검색 조건
{ $set: { name: 'john' } }, // 업데이트할 필드 및 값
{ new: true }, // 업데이트된 문서를 반환할지 여부 (기본값은 false)
)
Delete
const user = await userModel.deleteMany({ age: {$gte: 18}}) // 나이가 18세 이상인 것을 전부 삭제
const user = await userModel.deleteOne({name: 'tony'}) // name 이 tony 인 첫번째 document하나 삭제
const user = await userModel.findOneAndDelete({ name: 'tony'}) // parameteres 에 조건에 해당하는 document 1개 삭제
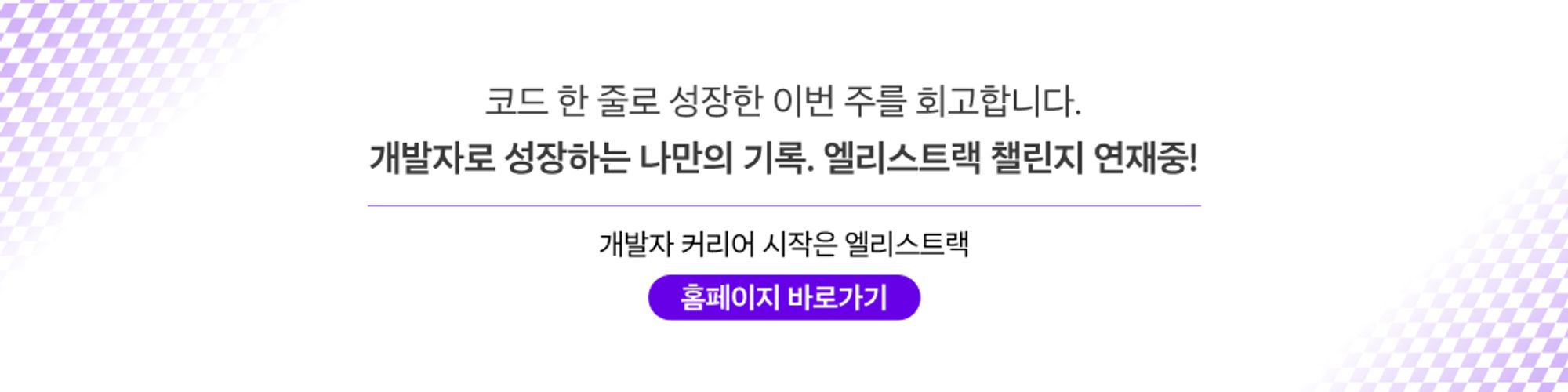
'Node.js' 카테고리의 다른 글
[node.js] JWT + Cookie 학습하기 (0) | 2024.02.10 |
---|
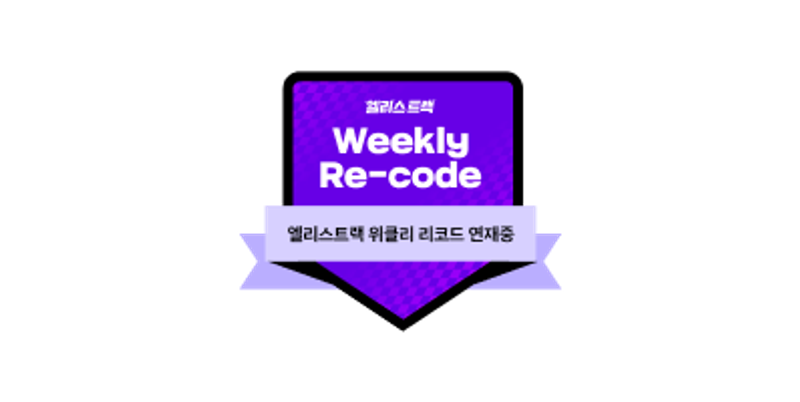
Mongodb 란?
- DataBase(하나 이상의 Collection을 가질 수 있는 저장소) →
Collection( 하나이상의 Document가 저장되는 공간) →
Document(저장되는 자료 SQL에서 row와 유사하지만 구조제약 없이 유연하게 저장가능) - Mongodb 는 비정형화된 데이터들을 저장하는 데이터베이스입니다.
- NoSQL 중에서 자주 사용하는 DB 이며 512MB 까지는 무료로 사용할 수 있습니다.
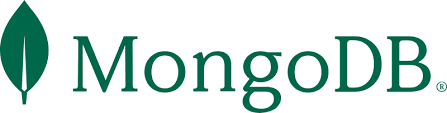
Mongoose 란?
- Mongoose 는 Mongodb 에서 schema를 보다 쉽게 관리할 수 있으며 Mongodb와의 연결 상태를 관리해주며 Populate를 사용하게 해주는 ODM ( Object Data Mapping) 입니다.
- Mongoose 를 사용하기 위해서는 패키지를 설치하여 사용합니다.
npm install mongoose
oryarn add mongoose
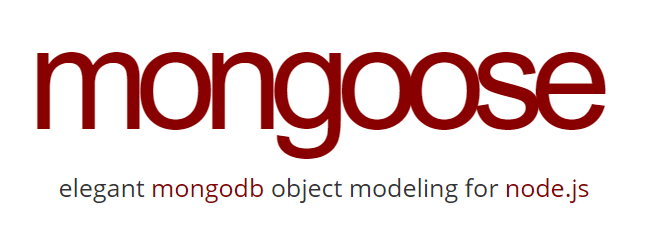
Mongoose 사용법
Mongoose 를 아래에 스키마와 모델을 생성하였다고 생각하고 CRUD 작업을 해보겠습니다.
const mongoose = require('mongoose');
const userSchema = mongoose.Schema({
name: String,
age: Number
})
const userModel = mongoose.model('users',userSchema);
Create (생성)
// 객체 생성을 통한 document 생성
const users = new userModel({ name:'tony', age: 23 })
await users.save();
// create 메서드 사용하여 document 생성
userModel.create({name: 'tony', age: 23})
Read (조회)
const users = await userModel.find({}); // 해당 collection 모두 조회
const user = await userModel.findOne({name: 'tony'}) // 해당 collection 에서 name 이 tony를 조회
const user = await userModel.findById(id) // _id를 기준으로 하나의 document를 반환
Update (변경)
const user = await userModel.update({name: 'tony'}, {name: 'william'}) // name이 tony 인 것을 wiliiam으로 변경!!
const user = await userModel.findByIdUpdate(id, {name: 'tony'}) // id에 해당하는 document를 찾아 name을 tony로 변경
const user = await userModel.findOneAndUpdate({ name: 'tony' }, // 검색 조건
{ $set: { name: 'john' } }, // 업데이트할 필드 및 값
{ new: true }, // 업데이트된 문서를 반환할지 여부 (기본값은 false)
)
Delete
const user = await userModel.deleteMany({ age: {$gte: 18}}) // 나이가 18세 이상인 것을 전부 삭제
const user = await userModel.deleteOne({name: 'tony'}) // name 이 tony 인 첫번째 document하나 삭제
const user = await userModel.findOneAndDelete({ name: 'tony'}) // parameteres 에 조건에 해당하는 document 1개 삭제
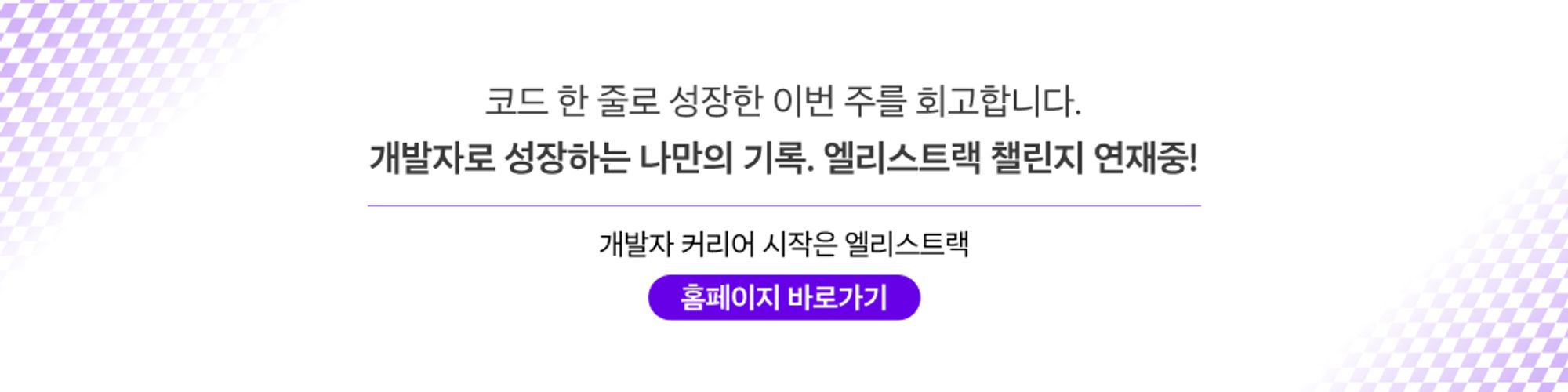
'Node.js' 카테고리의 다른 글
[node.js] JWT + Cookie 학습하기 (0) | 2024.02.10 |
---|